In this post, I will be regularly posting useful Python programming tips.
These tips will be organized in different categories, in order to make it easier to read it.
Hello World in Python
The below command, prints a “Hello World” message from within a Python script:
print("Hello World from Python")
Code Indentation in Python
In many programming languages, code indentation plays only a formatting role, however, in Python, code indentation is used for setting code blocks. Check for example the below if…else statement:
#if...elif...else example a=200 b=200 if a>b: print("a is greater than b") elif a==b: print("a and b are equal") else: print("b is greater than a")
As you can see, within each if, elif and else code blocks, I include one command in right indent.
This is an example of how code indentation is used in Python.
How to use Visual Studio Code for Python Development
Among the many IDE options you have for programming in Python, you can also use Visual Studio Code, which is a free IDE by Microsoft that supports developing in many programming languages.
So, after you have installed Visual Studio Code, you can find a free Python extension from its marketplace, install the extension and that’s it, you are ready to start developing in Python. In my setup, when developing in Python within Visual Studio Code, I use Microsoft’s Python extension.
In order of course to be able to run a Python script, you also need to install Python in your environment. You can find the available Python installers here.
Comments in Python
To comment a line of code in Python, you just need to use the # sign. Here’s an example:
#This is comment in Python
How to Easily Split a String in Python
In Python, you can split a string very easily. Check the below example:
string="Programming in Python" splitString=string.split() print(splitString) print(splitString[0]) print(splitString[1]) print(splitString[2])
Read also: Main Data Structures in Python
Learn More about Python Programming and SQL Server Data Access
Enroll to our online course “Working with Python on Windows and SQL Server Databases” with an exclusive discount, and get started with Python data access programming for SQL Server databases, fast and easy!
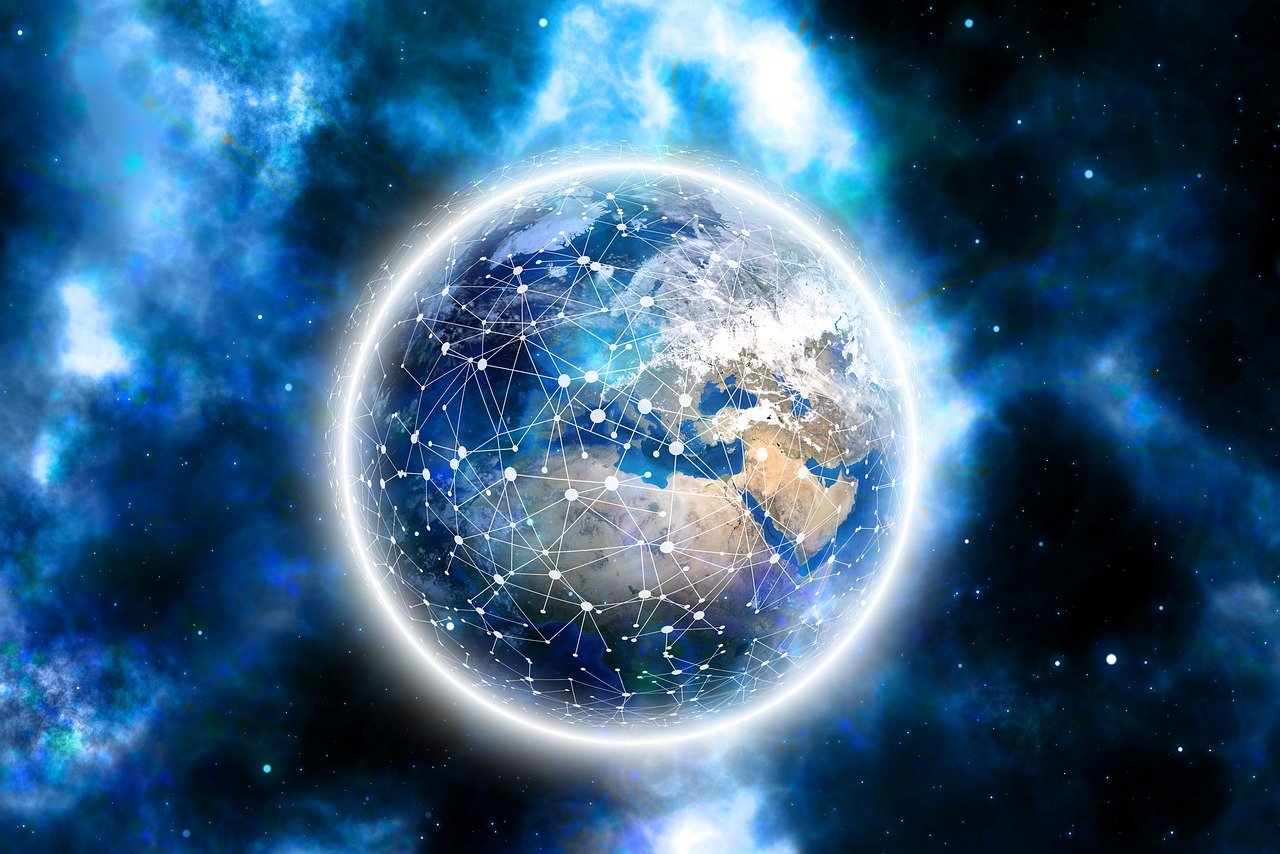
Get Started with Programming Fast and Easy – Enroll to the Online Course!
Check our online course “Introduction to Computer Programming for Beginners” (special limited-time discount included in link).
Learn the philosophy and main principles of Computer Programming and get introduced to C, C++, C#, Python, Java and SQL.
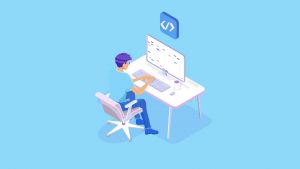
Featured Online Courses:
- Working with Python on Windows and SQL Server Databases
- Introduction to Computer Programming for Beginners
- AI Essentials: A Beginner’s Guide to Artificial Intelligence
- Human-AI Synergy: Teams and Collaborative Intelligence
- SQL Server 2022: What’s New – New and Enhanced Features
- Introduction to Azure Database for MySQL
- Boost SQL Server Database Performance with In-Memory OLTP
- Introduction to Azure SQL Database for Beginners
- Essential SQL Server Administration Tips
- SQL Server Fundamentals – SQL Database for Beginners
- Essential SQL Server Development Tips for SQL Developers
- .NET Programming for Beginners – Windows Forms with C#
- SQL Server 2019: What’s New – New and Enhanced Features
- Entity Framework: Getting Started – Complete Beginners Guide
- Data Management for Beginners – Main Principles
- A Guide on How to Start and Monetize a Successful Blog
Read Also:
- Python Data Access Fundamentals
- IndexError: list index out of range – How to Resolve it in Python
- How to Connect to SQL Server Databases from a Python Program
- How to Run the SQL Server BULK INSERT Command from Within a Python Program
- Working with Python on Windows and SQL Server Databases (Course Preview)
- IndentationError: expected an indented block in Python – How to Resolve it
- How to Resolve: [IM002] [Microsoft][ODBC Driver Manager] Data source name not found and no default driver specified (0) (SQLDriverConnect)
- Main Data Structures in Python
- How to Read a Text File in Python – Live Demo
- Missing parentheses in call to ‘print’. did you mean print(…) – How to Resolve in Python
- How to Write to a Text File from a C++ Program
- How to Establish a Simple Connection from a C# Program to SQL Server
- The timeout period elapsed prior to obtaining a connection from the pool
- Closing a C# Application (including hidden forms)
- Changing the startup form in a C# project
- Using the C# SqlParameter Object for Writing More Secure Code
- Cannot implicitly convert type ‘string’ to ‘System.Windows.Forms.DataGridViewTextBoxColumn
Check our online courses!
Check our eBooks!
Subscribe to our YouTube channel!
Subscribe to our newsletter and stay up to date!
Rate this article:
Reference: SQLNetHub.com (https://www.sqlnethub.com)
© SQLNetHub
Artemakis Artemiou, a distinguished Senior Database and Software Architect, brings over 20 years of expertise to the IT industry. A Certified Database, Cloud, and AI professional, he earned the Microsoft Data Platform MVP title for nine consecutive years (2009-2018). As the founder of SQLNetHub and GnoelixiAI Hub, Artemakis is dedicated to sharing his knowledge and democratizing education on various fields such as: Databases, Cloud, AI, and Software Development. His commitment to simplicity and knowledge sharing defines his impactful presence in the tech community.